この記事の目次
SalesforceのContinuationクラスは何ができるの?
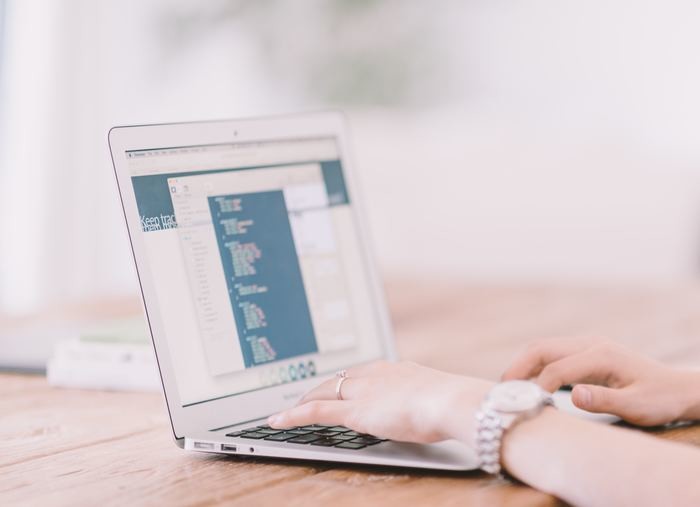
- Visualforceの場合、Spring’15(APIバージョン34.0)以降
- Lightning Componentの場合、Summer’19(APIバージョン46.0)以降
Visualforceページから非同期コールアウトを実行する方法
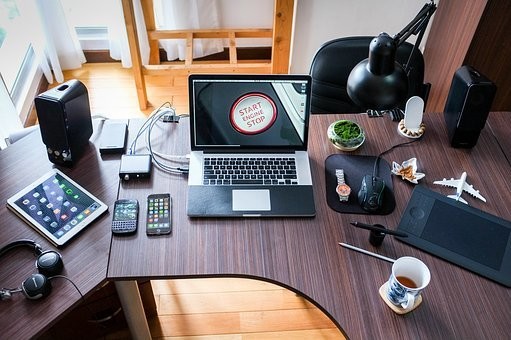
【Step1】ApexのControllerクラスを実装する
ApexのControllerクラスにアクションメソッドとコールバックメソッドを実装します。public with sharing class TestController {
// リクエストラベル
public String requestLabel;
// Visualforceページに表示する結果のプロパティ
public String result { get; set;}
private static final String END_POINT = 'https://XXXX.com/address';
// アクションメソッド
public Object startRequest() {
// Continuationクラスの引数にタイムアウト(秒)を設定。120秒まで設定可能。
Continuation con = new Continuation(60);
// コールバックメソッド名を指定
con.continuationMethod = 'runResponse';
HttpRequest req = new HttpRequest();
req.setMethod('GET');
req.setEndpoint(END_POINT);
// ContinuationクラスにHTTPリクエストを追加し、リクエストラベルに代入
this.requestLabel = con.addHttpRequest(req);
// Continuationクラスを返却
return con;
}
// コールバックメソッド
public Object runResponse() {
// リクエストラベルを使って、レスポンスを取得する
HttpResponse response = Continuation.getResponse(this.requestLabel);
// Visualforceページに表示される結果にレスポンスを格納
this.result = response.getBody();
// 呼び出し元のVisualforceページを再描画するためにnullを返却
return null;
}
}
【Step2】VisualForceページを実装する
VisualForceページにControllerクラスのアクションメソッドを呼び出す処理を実装します。<apex:page controller=""TestController"" showChat=""false"" showHeader=""false"">
<apex:form >
<!-- 「Start」ボタンを押すと、アクションメソッドを実行. -->
<apex:commandButton action=""{!startRequest}""
value=""Start"" reRender=""result""/>
</apex:form>
<!-- Webサービスの実行結果を表示. -->
<apex:outputText value=""{!result}"" />
</apex:page>
Lightning Componentから非同期コールアウトを実行する方法
SalesforceのLightning Componentを利用して、Continuationクラスでの非同期コールアウト実行方法を実装例とともに紹介します。【Step1】Apexクラスを実装する
Apexクラスにアクションメソッドとコールバックメソッドを実装します。public with sharing class TestApex {
private static final String END_POINT = 'https://XXXX.com/address';
// アクションメソッド
// @AuraEnabled(continuation=true cacheable=true)を設定
@AuraEnabled(continuation=true cacheable=true)
public static Object startRequest() {
// Continuationクラスの引数にタイムアウト(秒)を設定
Continuation con = new Continuation(60);
// コールバックメソッド名を指定
con.continuationMethod = 'runResponse';
HttpRequest req = new HttpRequest();
req.setMethod('GET');
req.setEndpoint(END_POINT);
// ContinuationクラスにHTTPリクエストを追加
con.addHttpRequest(req);
// Continuationクラスを返却
return con;
}
// コールバックメソッド
// Continuationクラスのコールバックメソッドには@AuraEnabled(cacheable=true)を付与
@AuraEnabled(cacheable=true)
public static Object runResponse(List<String> labels, Object state) {
// 0番目のlabelsをキーにして、レスポンスを取得する
HttpResponse response = Continuation.getResponse(labels[0]);
// 呼び出し元のLightning Componentにレスポンスを返却
return response.getBody();
}
}
【Step2】JavaScriptを実装する
JavaScriptに「@salesforce/apexContinuation」でApexクラスを呼び出すよう実装します。import { LightningElement, track } from 'lwc';
// 「@salesforce/apexContinuation」でApexクラスを呼び出す
import startRequest from '@salesforce/apexContinuation/TestApex.startRequest';
export default class ContinuationSample extends LightningElement {
callWebService() {
startRequest().then(result => {
this.imperativeContinuation = result;
}).catch(error => {
this.imperativeContinuation = error;
});
}
get formattedWebServiceResult() {
return JSON.stringify(this.imperativeContinuation);
}
}
【Step3】HTMLを実装する
HTMLにWebサービスを呼び出すJavaScriptを実行する処理を実装します。<template>
<lightning-button label=""Start"" onclick={callWebService}></lightning-button>
<div>
result: {formattedWebServiceResult}
</div>
</template>
Continuationクラスで非同期コールアウトを実装しよう
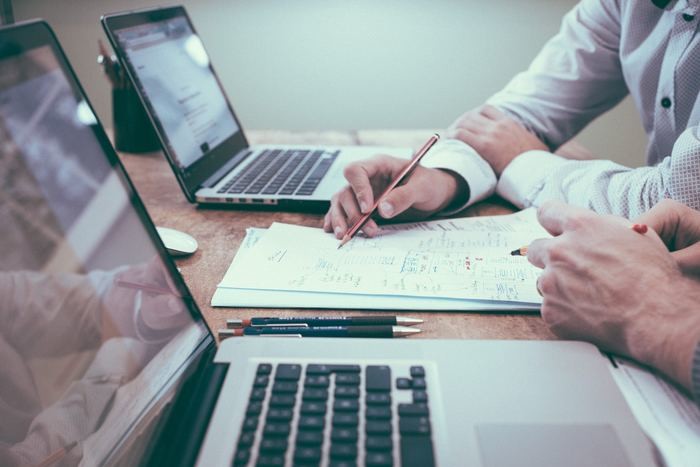
この記事の監修者・著者
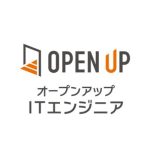
-
未経験からITエンジニアへのキャリアチェンジを支援するサイト「キャリアチェンジアカデミー」を運営。これまで4500人以上のITエンジニアを未経験から育成・排出してきました。
・AWS、salesforce、LPICの合計認定資格取得件数:2100以上(2023年6月時点)
・AWS Japan Certification Award 2020 ライジングスター of the Year 受賞
最新の投稿
- 2024年3月26日キャリア・転職保安職(自衛官・警察・消防官など)に向いている人の性格・特徴ランキング【現役保安職(自衛官・警察・消防官など)36人が回答】
- 2024年3月26日キャリア・転職保安職(自衛官・警察・消防官など)に必要なスキルランキング&スキルアップの方法とは?【現役保安職(自衛官・警察・消防官など)36人が回答】
- 2024年3月26日キャリア・転職クリエイター職(ライター・デザイナー・編集)に向いている人の性格・特徴ランキング【現役クリエイター職(ライター・デザイナー・編集)64人が回答】
- 2024年3月26日キャリア・転職クリエイター職(ライター・デザイナー・編集)に必要なスキルランキング&スキルアップの方法とは?【現役クリエイター職(ライター・デザイナー・編集)64人が回答】